What Is models.CASCADE
When working with databases in web development, it is common to come across the term models.CASCADE. This is a concept widely used in the Django web framework, which is a popular choice for building web applications. Understanding what models.CASCADE means and how it is implemented is important for anyone working with Django or other similar frameworks.
Key Takeaways:
- models.CASCADE is a feature in Django that allows for automatic cascading deletion of related database objects.
- It ensures that when a parent object is deleted, its related objects are also deleted to maintain data integrity.
- models.CASCADE can be used in Django’s foreign key and one-to-one relationships.
- It is a convenient way to manage database relationships and prevent orphaned records.
How models.CASCADE Works
models.CASCADE is a parameter or option that can be set when defining a foreign key or one-to-one relationship in Django models. When a parent object is deleted, and models.CASCADE is specified, all the related child objects are automatically deleted by Django. This ensures that no orphaned records are left in the database and helps maintain data integrity.
**For example**, consider a scenario where you have a blog application with two models: User and Post. Each post is associated with a user using a foreign key relationship. If a user decides to delete their account, you can use models.CASCADE to delete all their associated posts, preventing any data inconsistencies.
Implementation Example
Let’s look at an example of how models.CASCADE is implemented in Django. Suppose we have two models: Author and Book. Each book is associated with an author using a foreign key relationship. We want to ensure that if an author is deleted, all their associated books are also removed from the database.
The models in Django could be defined as follows:
Model | Code |
---|---|
Author |
|
Book |
|
Using models.CASCADE in the ForeignKey field of the Book model ensures that when an author is deleted, all the associated books are also deleted automatically.
Advantages of models.CASCADE
- **One of the advantages** of using models.CASCADE is that it simplifies the management of related database records. You don’t have to manually delete the associated child records when deleting a parent object, as Django takes care of it.
- **Another advantage** is that models.CASCADE helps prevent orphaned records in the database. It ensures that there are no related child records left behind without a parent and maintains data consistency.
Conclusion
In conclusion, models.CASCADE is a helpful feature in Django that allows for automatic cascading deletion of related objects in a database. By setting models.CASCADE on the foreign key or one-to-one relationship, you can ensure that when a parent object is deleted, its related objects are also removed, maintaining data integrity and preventing orphaned records. Understanding and appropriately implementing models.CASCADE are essential skills for any Django developer.
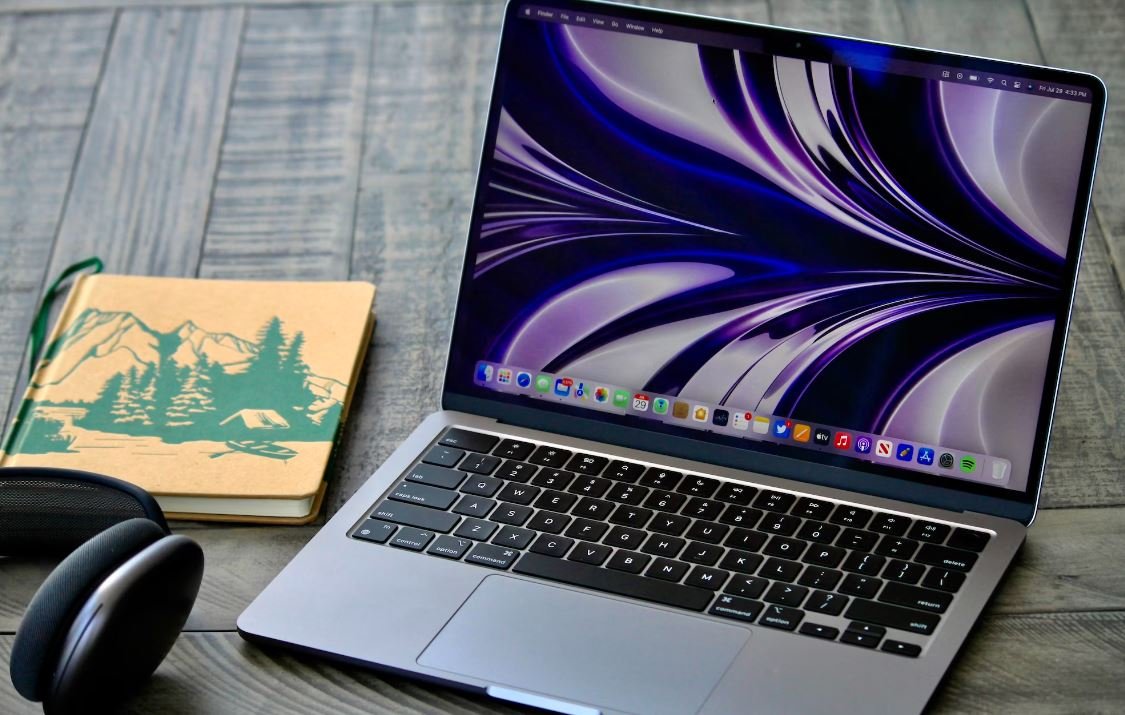
Common Misconceptions
1. Models.CASCADE is automatic for related fields
One common misconception about models.CASCADE in Django is that it is automatically applied to related fields. However, this is incorrect. Models.CASCADE is a parameter that needs to be explicitly set when defining the relationship between models.
- Setting models.CASCADE is not automatic
- Models should specify models.CASCADE when defining relationships
- Models.CASCADE is not the default behavior for related fields
2. Using models.CASCADE will delete all related objects
Another common misconception is that using models.CASCADE will delete all related objects when the parent object is deleted. While models.CASCADE does trigger the deletion of related objects, it does not mean that all related objects will be deleted in all scenarios.
- models.CASCADE triggers deletion of related objects
- Related objects may not be deleted in all scenarios
- Deleting a parent object can have different outcomes depending on the implementation
3. models.CASCADE eliminates the need to handle related object deletion manually
There is a misconception that models.CASCADE eliminates the need to handle related object deletion manually. While models.CASCADE takes care of the basic deletion of related objects, there may be additional logic or steps required depending on the specific requirements of the application.
- models.CASCADE handles basic related object deletion
- Additional logic may be needed for specific requirements
- The implementation might require custom deletion handling
4. models.CASCADE can only be used with foreign key relationships
It is commonly believed that models.CASCADE can only be used with foreign key relationships. However, this is a misconception. models.CASCADE can also be used with other types of relationships, such as OneToOneField or ManyToManyField.
- models.CASCADE can be used with various relationship types
- Not limited to foreign key relationships
- Can be used with OneToOneField and ManyToManyField, among others
5. models.CASCADE is the only option for handling related object deletion
Lastly, there is a misconception that models.CASCADE is the only option for handling related object deletion in Django. While models.CASCADE is a commonly used option, Django provides other options like models.PROTECT, models.SET_NULL, and models.SET_DEFAULT, among others.
- models.CASCADE is not the only option for handling deletion
- Other options like models.PROTECT, models.SET_NULL, etc., exist
- Different options provide flexibility for different scenarios
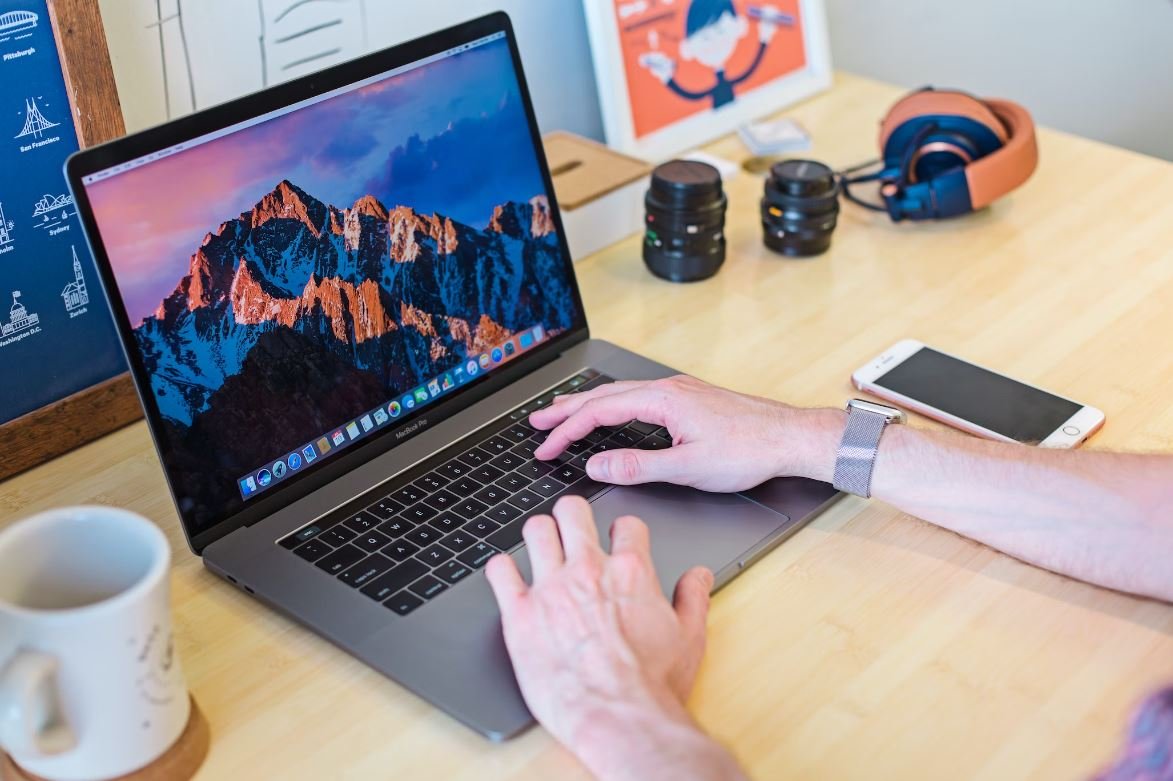
What Is models.CASCADE?
models.CASCADE is a database operation that ensures the integrity and consistency of data in a relational database system. It is a key concept in database management that refers to the behavior of related database entities when the referenced entity is deleted or updated. In this article, we will explore various scenarios and examples to understand the use and significance of models.CASCADE.
Deleting a User:
Consider a scenario where users have various associated entities, such as posts, comments, and likes. When a user is deleted, using models.CASCADE ensures that all associated entities are also deleted from the database. This prevents orphaned data and maintains the integrity of the system.
User ID | Username | |
---|---|---|
1 | JohnDoe | johndoe@email.com |
2 | JaneSmith | janesmith@email.com |
Deleting a Post:
Deleting a post using models.CASCADE ensures that all associated comments, likes, and share records are also deleted. This cascading effect simplifies data management and prevents inconsistencies within the database.
Post ID | Title | Content |
---|---|---|
1 | Introduction to models.CASCADE | An overview of the models.CASCADE feature in database systems. |
2 | Advanced SQL Techniques | A deep dive into complex SQL queries and optimizations. |
Updating a Category:
When updating the category of an entity, such as a post or product, models.CASCADE ensures that all related entities are updated accordingly. This reduces manual effort and maintains consistent categorization throughout the system.
Product ID | Name | Category |
---|---|---|
1 | iPhone 12 | Smartphones |
2 | Macbook Pro | Laptops |
Deleting a Product:
When a product is deleted, using models.CASCADE ensures that all associated inventory records, orders, and customer reviews are also removed. This simplifies data management and ensures that redundant information is not left behind in the database.
Product ID | Name | Price |
---|---|---|
1 | Canon EOS R5 | $3,499 |
2 | Sony A7 III | $1,999 |
Updating an Order:
When an order is updated, such as changing the shipping address or adding/removing items, models.CASCADE ensures that all associated details, including customer information and payment records, are also updated accordingly. This maintains the accuracy and consistency of order information within the system.
Order ID | Customer Name | Shipping Address |
---|---|---|
1 | John Doe | 123 Main Street |
2 | Jane Smith | 456 Elm Avenue |
Deleting a Category:
Deleting a category using models.CASCADE ensures that all associated entities, such as posts or products, are also deleted. This eliminates the need to manually remove dependencies and maintains a clean and organized database structure.
Category ID | Name | Description |
---|---|---|
1 | Books | Novels, non-fiction, textbooks, and more. |
2 | Electronics | Computers, TVs, smartphones, and appliances. |
Updating a Comment:
When a user updates a comment associated with a post or any other entity, models.CASCADE ensures that the updated comment is reflected everywhere. This ensures consistency in the displayed content and prevents confusion or outdated information.
Comment ID | Content | User |
---|---|---|
1 | Great article! | JohnDoe |
2 | Very informative. | JaneSmith |
Deleting a Tag:
Deleting a tag using models.CASCADE ensures that all associated posts or other tagged entities are also updated accordingly. This prevents inconsistent tag references and maintains the integrity of data within the system.
Tag ID | Name |
---|---|
1 | Technology |
2 | Science |
Updating a Like:
When a user updates their like on a post or any other entity, models.CASCADE ensures that the like count and associated information are updated accordingly. This provides an accurate representation of user preferences and prevents inconsistencies within the system.
Like ID | User | Entity Liked |
---|---|---|
1 | JohnDoe | Post ID: 1 |
2 | JaneSmith | Product ID: 2 |
Conclusion:
models.CASCADE is a powerful mechanism in database management that ensures the integrity and consistency of data when related entities are deleted or updated. By automatically cascading changes, it simplifies data management, prevents orphaned records, and maintains a clean and reliable database structure. Understanding models.CASCADE is essential for developing robust and efficient database systems.
Frequently Asked Questions
What is models.CASCADE in Django?
models.CASCADE is a parameter in Django that specifies the behavior to follow when the referenced object is deleted. If models.CASCADE is set and a referenced object is deleted, Django will also delete the objects that have a foreign key to the deleted object.
How do you define a foreign key with models.CASCADE?
To define a foreign key with models.CASCADE, you can use the ‘on_delete=models.CASCADE’ parameter when creating the foreign key field in your model.
What happens if models.CASCADE is not specified?
If models.CASCADE is not specified, Django will raise a ‘TypeError’ during the creation of the foreign key field. It is required to specify an on_delete behavior for the foreign key field.
Can you use other on_delete behaviors with models.CASCADE?
No, models.CASCADE can only be used as the on_delete behavior. Other options for on_delete include models.PROTECT, models.SET_NULL, models.SET_DEFAULT, models.SET(), and models.DO_NOTHING.
How does models.CASCADE affect related objects?
When models.CASCADE is used, deleting a referenced object will also delete all related objects that have a foreign key to the deleted object. This can be useful for maintaining referential integrity in the database.
Can models.CASCADE cause data loss when deleting objects?
Yes, using models.CASCADE without caution can result in unintended data loss. It is recommended to back up your database and thoroughly test your application before using models.CASCADE.
What is the default on_delete behavior if models.CASCADE is not set?
If models.CASCADE is not set, the default on_delete behavior is models.CASCADE. This ensures that related objects are also deleted when the referenced object is deleted.
Can models.CASCADE be used with many-to-many relationships?
No, models.CASCADE is not applicable to many-to-many relationships in Django. Instead, you can use models.SET_NULL, models.SET_DEFAULT, or models.SET() as on_delete behaviors.
Are there any risks or considerations when using models.CASCADE?
Using models.CASCADE without understanding its implications can lead to accidental data loss or inconsistencies. It is important to carefully plan and test your data relationships and take appropriate precautions.
Can you update the on_delete behavior of an existing foreign key?
No, you cannot directly update the on_delete behavior of an existing foreign key. Instead, you should create a new migration to modify the foreign key and specify the desired on_delete behavior.